Here are my 2 cents worth on MVC pattern.
MVC is an architectural pattern used in software engineeering. Successful use of the pattern isolates business logic from user interface considerations, resulting in an application where it is easier to modify either the visual appearance of the application or the underlying business rules without affecting the other.
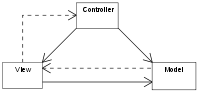
MVC Pattern
The whole Navitaire application is based on the MVC or Model View Controller Pattern; also known as MVP or Model View Presenter design pattern.
Model
The Model is responsible for managing application data and state, and communicating with a server. When an operation is performed on a model that changes the data or state of an application, the model notifies all of its consumers.
View
The View represents the presentation layer of an application. A view does not necessarily refer to a Windows form or a Web page and may not be visual at all. For example, a Web service or voice interface can be different views of the application that present the application data to the user.
Controller
The Controller provides validation, security enforcement and application workflow logic. For each user operation defined in an application there should be a controller method that is invoked that validates the data and security before invoking method calls on a model object. Logic related to application workflow can also be contained in the controller and may be dependent upon the active view.
As usual don't forget to leave comments. Thanks.